Результат виглядає ось так...
Ці коди працюють разом, щоб створити повністю функціональну та іграбельну гру.
Цей код створює гру "Крестики-Нолики", в яку ви можете грати проти комп'ютера.
HTML
HTML-структура створює ігрове поле та простий інтерфейс для користувача.
Tic Tac Toe
Ваш хід (X)
Перезапустити гру
``` > CSS CSS додає стилізацію, включаючи градієнтний фон, ефекти при наведенні та анімації, щоб гра виглядала привабливо.
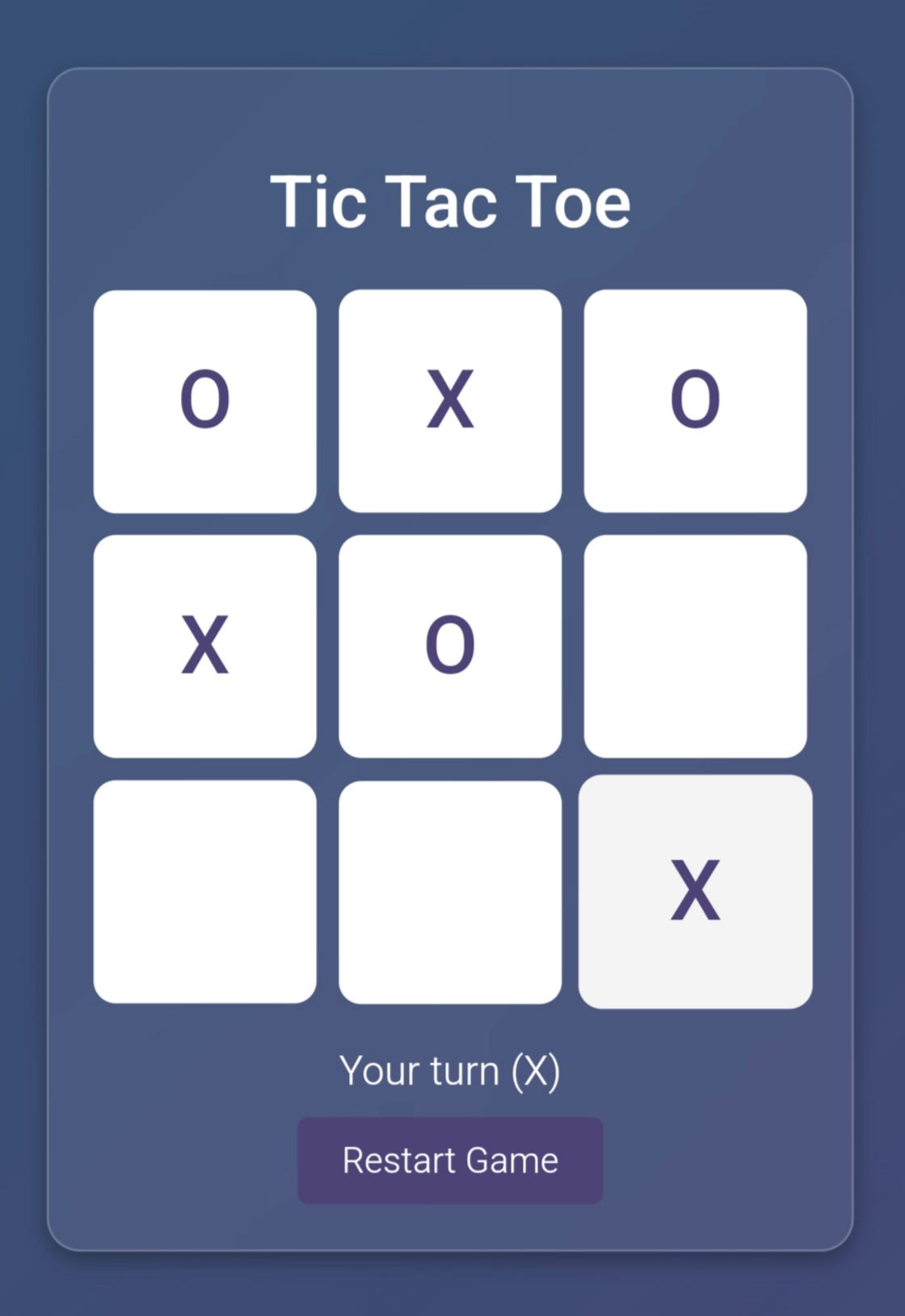
_Результат виглядає ось так..._
Ці коди працюють разом, щоб створити повністю функціональну та іграбельну гру.
Цей код створює гру "Крестики-Нолики", в яку ви можете грати проти комп'ютера.
> HTML
HTML-структура створює ігрове поле та простий інтерфейс для користувача.
Tic Tac Toe
Ваш хід (X)
Перезапустити гру
``` > CSS CSS додає стилізацію, включаючи градієнтний фон, ефекти при наведенні та анімації, щоб гра виглядала привабливо.
body {
font-family: 'Arial', sans-serif;
background: linear-gradient(135deg, #2b5876, #4e4376);
color: white;
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
overflow: hidden;
}
.container {
text-align: center;
box-shadow: 0 4px 10px rgba(0, 0, 0, 0.3);
border-radius: 15px;
padding: 20px;
background-color: #ffffff10;
backdrop-filter: blur(8px);
border: 1px solid rgba(255, 255, 255, 0.2);
}
h1 {
margin-bottom: 20px;
}
#game {
display: grid;
grid-template-columns: repeat(3, 100px);
gap: 10px;
margin: 0 auto 20px;
}
.cell {
width: 100px;
height: 100px;
background-color: #fff;
color: #4e4376;
border-radius: 10px;
display: flex;
align-items: center;
justify-content: center;
font-size: 36px;
font-weight: bold;
cursor: pointer;
transition: transform 0.2s, background-color 0.3s;
}
.cell:hover {
background-color: #f4f4f4;
transform: scale(1.05);
}
.cell.taken {
pointer-events: none;
}
#status {
font-size: 18px;
margin: 10px 0;
}
#restart {
padding: 10px 20px;
font-size: 16px;
background-color: #4e4376;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s;
}
#restart:hover {
background-color: #6a6180;
}
JavaScript
JavaScript обробляє логіку гри та робить комп'ютера складним суперником за допомогою алгоритму мінімакс.
const cells = document.querySelectorAll('.cell');
const statusText = document.getElementById('status');
const restartBtn = document.getElementById('restart');
let board = ['', '', '', '', '', '', '', '', ''];
let isComputerX = true; // Чередує роль комп'ютера між X та O
let currentPlayer, computerPlayer;
const winningCombos = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6],
];
function initializeGame() {
currentPlayer = isComputerX ? 'O' : 'X';
computerPlayer = isComputerX ? 'X' : 'O';
board = ['', '', '', '', '', '', '', '', ''];
cells.forEach(cell => {
cell.textContent = '';
cell.classList.remove('taken');
});
statusText.textContent = `Ваш хід (${currentPlayer})`;
if (currentPlayer === computerPlayer) {
setTimeout(bestMove, 500);
}
}
function checkWinner(player) {
return winningCombos.some(combo =>
combo.every(index => board[index] === player)
);
}
function isDraw() {
return board.every(cell => cell !== '');
}
function bestMove() {
let bestScore = -Infinity;
let move;
for (let i = 0; i < board.length; i++) {
if (board[i] === '') {
board[i] = computerPlayer;
let score = minimax(board, 0, false);
board[i] = '';
if (score > bestScore) {
bestScore = score;
move = i;
}
}
}
board[move] = computerPlayer;
cells[move].textContent = computerPlayer;
cells[move].classList.add('taken');
if (checkWinner(computerPlayer)) {
statusText.textContent = `Комп'ютер (${computerPlayer}) виграв!`;
endGame();
} else if (isDraw()) {
statusText.textContent = "Нічия!";
} else {
currentPlayer = currentPlayer === 'X' ? 'O' : 'X';
statusText.textContent = `Ваш хід (${currentPlayer})`;
}
}
function minimax(board, depth, isMaximizing) {
if (checkWinner(computerPlayer)) return 10 - depth; // Комп'ютер виграв
if (checkWinner(currentPlayer)) return depth - 10; // Гравець виграв
if (isDraw()) return 0; // Нічия
if (isMaximizing) {
let bestScore = -Infinity;
for (let i = 0; i < board.length; i++) {
if (board[i] === '') {
board[i] = computerPlayer;
let score = minimax(board, depth + 1, false);
board[i] = '';
bestScore = Math.max(score, bestScore);
}
}
return bestScore;
} else {
let bestScore = Infinity;
for (let i = 0; i < board.length; i++) {
if (board[i] === '') {
board[i] = currentPlayer;
let score = minimax(board, depth + 1, true);
board[i] = '';
bestScore = Math.min(score, bestScore);
}
}
return bestScore;
}
}
function handleCellClick(e) {
const cell = e.target;
const index = cell.dataset.index;
if (board[index] === '' && currentPlayer !== computerPlayer) {
board[index] = currentPlayer;
cell.textContent = currentPlayer;
cell.classList.add('taken');
if (checkWinner(currentPlayer)) {
statusText.textContent = `Ви (${currentPlayer}) виграли!`;
endGame();
} else if (isDraw()) {
statusText.textContent = "Нічия!";
} else {
currentPlayer = computerPlayer;
statusText.textContent = `Хід комп'ютера (${computerPlayer})`;
setTimeout(bestMove, 500);
}
}
}
function endGame() {
cells.forEach(cell => cell.classList.add('taken'));
}
restartBtn.addEventListener('click', () => {
isComputerX = !isComputerX; // Чередуємо ролі
initializeGame();
});
cells.forEach(cell => cell.addEventListener('click', handleCellClick));
initializeGame();
Коротко кажучи, HTML створює гру, CSS робить її привабливою, а JavaScript робить її функціональною!
Збережіть файли як index.html, styles.css і script.js, і перевірте в браузері.
Гра є адаптивною і підтримує як десктопні, так і мобільні пристрої, забезпечуючи плавний і приємний досвід.
— Коди від yellow hell…
Перекладено з: Code for tic-tac-toe game…(Noughts and crosses or XOX)…